Arduino Documentation Blog Entry
There are 4 tasks that will be explained in this page:
1.Input devices:
a.Interface a potentiometer analog input to maker UNO board and
measure/show its signal in serial monitor Arduino IDE.
b.Interface a LDR to maker UNO board and measure/show its signal in
serial monitor Arduino IDE
2.Output devices:
a. Interface 3 LEDs (Red, Yellow, Green) to maker UNO board and program
it to perform something (fade or flash etc)
b. Include the pushbutton on the MakerUno board to start/stop part 2.a.
above
For each of the tasks, I will describe:
1.The program/code that I have used and explanation of the code. The code is
in writable format (not an image).
2.The sources/references that I used to write the code/program.
3.The problems I encountered and how I fixed them.
4.The evidence that the code/program worked in the form of video of the
executed program/code.
Finally, I will describe:
5.My Learning reflection on the overall Arduino programming activities.
Input devices: Interface a potentiometer analog input to maker UNO board and measure/show its signal in serial monitor Arduino IDE.
1.Below are the code/program I have used and the explanation of the code.
Code/program in writeable format | Explanation of the code |
int sensorPin = A0;
int ledPin = 13;
int sensorValue = 0;
void setup() {
pinMode(sensorPin, INPUT);
pinMode(ledPin, OUTPUT);
}
void loop() {
sensorValue = analogRead(sensorPin);
digitalWrite(ledPin, HIGH);
delay(sensorValue);
digitalWrite(ledPin, LOW);
delay(sensorValue);
}
| int sensorPin = A0 - select the input pin for the potentiometer int ledPin = 13 - select the pin for the LED int sensorValue = 0 - variable to store the value coming from the sensor Void Setup pinMode(sensorPin, INPUT) - declare the sensorPin as an INPUT pinMode(ledPin, OUTPUT) - declare the ledPin as an OUTPUT Void Loop sensorValue = analogRead(sensorPin)- read the value from the sensor digitalWrite(ledPin, HIGH) - turn the ledPin on delay(sensorValue) - stop the program for <sensorValue> milliseconds digitalWrite(ledPin, LOW) - turn the ledPin off delay(sensorValue) - stop the program for for <sensorValue> milliseconds |
2. Below are the hyperlink to the sources/references that I used to write the
code/program.
3.Below are the problems I have encountered and how I fixed them.
Problem:
LED wont not light up when programme is running fine.
Solution:
LED was connected incorrectly, fixed it by switching the connection between the anode and the cathode
4.Below is the short video as the evidence that the code/program work.
Input devices: Interface a LDR to maker UNO board and measure/show its signal in serial monitor Arduino IDE:
1.Below are the code/program I have used and the explanation of the code
Code/program in writeable format | Explanation of the code |
int LDR = A0; int LDRvalue = 0; void setup() { Serial.begin(9600); } void loop() { LDRvalue = analogRead(LDR); Serial.println(LDR); delay(100)
}
| int LDR = A0 - select the input pin for the LDR int LDRValue = 0 - variable to store the value coming from the sensor Void Setup Serial.begin(9600) - etsablishes serial communication between the uno board and another device Void Loop LDRvalue = analogRead(LDR) - reads the input of pin and writes into an integer variable Serial.println(LDR) - print the value of the integer variable delay(100) - a delay of 100 milliseconds |
2. Below are the hyperlink to the sources/references that I used to write the
code/program.
3. Below are the problems I have encountered and how I fixed them.
Problem:
Initially the graph would not show up on the laptop screen even though the "serial plotter" icon has been pressed
Solution:
Had to rerun the code again and the "serial plotter" graph popped up
4. Below is the short video as the evidence that the code/program work
Output devices: Interface 3 LEDs (Red, Yellow, Green) to maker UNO board and program it to perform something (fade or flash etc)
1. Below are the code/program I have used and the explanation of the code.
Code/program in writeable format | Explanation of the code |
int animationSpeed = 0; void setup() { pinMode(13, OUTPUT); pinMode(12, OUTPUT); pinMode(11, OUTPUT); } void loop() { animationSpeed = 200 ; digitalWrite(13, HIGH); delay(animationSpeed); // Wait for animationSpeed millisconds (s) digitalWrite(13, LOW); delay(animationSpeed); // Wait for animationSpeed millisconds (s) digitalWrite(12, HIGH); delay(animationSpeed); // Wait for animationSpeed millisconds (s) digitalWrite(12, LOW); delay(animationSpeed); // Wait for animationSpeed millisconds (s) digitalWrite(11, HIGH); delay(animationSpeed); // Wait for animationSpeed millisconds (s) digitalWrite(11, LOW); delay(animationSpeed); // Wait for animationSpeed millisconds (s) } | int animationSpeed = 0 - defines animationSpeed as an integer variable Void Setup pinMode(13, OUTPUT) - declare the Pin 13 as an OUTPUT pinMode(12, OUTPUT) - declare the Pin 12 as an OUTPUT pinMode(11, OUTPUT) - declare the Pin 11 as an OUTPUT Void loop animationSpeed = 200 ; digitalWrite(13, HIGH) - turn on led at pin 13 delay(animationSpeed) - delay of 200 seconds before the next action digitalWrite(13, LOW) - turn off led at pin 13 delay(animationSpeed) - delay of 200 seconds before the next action digitalWrite(12, HIGH) - turn on led at pin 12 delay(animationSpeed) - delay of 200 seconds before the next action digitalWrite(12, LOW) - turn off led at pin 12 delay(animationSpeed) - delay of 200 seconds before the next action digitalWrite(11, HIGH) - turn on led at pin 11 delay(animationSpeed) - delay of 200 seconds before the next action digitalWrite(11, LOW) - turn off led at pin 11 delay(animationSpeed) - delay of 200 seconds before the next action |
2. Below are the hyperlink to the sources/references that I used to write the
code/program.
3.Below are the problems I have encountered and how I fixed them.
Problem:
LED wont not light up when programme is running fine.
Solution:
LED was connected incorrectly, fixed it by switching the connection between the anode and the cathode
4.Below is the short video as the evidence that the code/program work.
Output devices: Include pushbutton to start/stop the previous task
1.Below are the code/program I have used and the explanation of the code.
Code/program in writeable format | Explanation of the code |
int animationSpeed = 0; void setup() { Serial.begin(9600); pinMode(13, OUTPUT); pinMode(12, OUTPUT); pinMode(11, OUTPUT); pinMode(2, INPUT_PULLUP); } void loop() { int sensorVal = digitalRead(2); Serial.println(sensorVal); if (sensorVal == HIGH) { animationSpeed = 200 ; digitalWrite(13, HIGH); delay(animationSpeed); // Wait for animationSpeed millisconds (s) digitalWrite(13, LOW); delay(animationSpeed); // Wait for animationSpeed millisconds (s) digitalWrite(12, HIGH); delay(animationSpeed); // Wait for animationSpeed millisconds (s) digitalWrite(12, LOW); delay(animationSpeed); // Wait for animationSpeed millisconds (s) digitalWrite(11, HIGH); delay(animationSpeed); // Wait for animationSpeed millisconds (s) digitalWrite(11, LOW); delay(animationSpeed); // Wait for animationSpeed millisconds (s) } else { digitalWrite (13, LOW); digitalWrite (12, LOW); digitalWrite (11, LOW); } } | int animationSpeed = 0 - defines animationSpeed as an integer variable Void Setup Serial.begin(9600) - etsablishes serial communication between the uno board and another device pinMode(13, OUTPUT) - declare the Pin 13 as an OUTPUT pinMode(12, OUTPUT) - declare the Pin 12 as an OUTPUT pinMode(11, OUTPUT) - declare the Pin 11 as an OUTPUT pinMode(2, INPUT_PULLUP) Void Loop int sensorVal = digitalRead(2) - reads the input of pin 2 and writes into a integer variable. When the button is pressed, the status will change to low and releasing it will change to high. Serial.println(sensorVal) - prints the value of sensorVal when the button is pressed if (sensorVal == HIGH) - If sensorVal status is HIGH, all the pin will be HIGH, else, it will be LOW |
2. Below are the hyperlink to the sources/references that I used to write the code/program.
3. Below are the problems I have encountered and how I fixed them.
Problem:
1.LED wont not light up when programme is running fine.
2. The programmable button will not work even though the programme is running fine
Solution:
1. LED was connected incorrectly, fixed it by switching the connection between the anode and the cathode
2. The bracket in the code was not properly coded in the code, had to properly code the bracket in and button worked fine
4. Below is the short video as the evidence that the code/program work.
Below is my Learning Reflection on the overall Arduino Programming activities.
When Arduino was first introduced to me, I was very overwhelmed and confused as I have never code in my life and I never understood why would I need to know how to code as a chemical engineer. So as Mr Chua talked more about Arduino, he also exposed my class to the uno maker kit. At first glance, it was just some ordinary box. As I opened the box, there was so many components that looked very interesting to me. There was the jumper cable, bread board, potentiometer, couple of LEDs and resistors, finally the uno maker board. I was very intrigued by the uno maker board as when you connect the maker board to your laptop, it makes a short ringtone which I thought was pretty cool.
After learning about the components in the uno maker kit, we proceeded to install the coding software which was the Arduino IDE. One thing I found IDE interesting was that there was built-in code examples that we could use, so it made coding much more simpler and faster. I also asked around my peers which are from the School of Computing in SP, however, they have no idea what was Arduino was. Hence, I believe that Arduino was probably made for engineers.
After playing around with the examples in Arduino IDE, I proceeded on to the learning packages which was also part of the pre-practical assignment for the Arduino practical. These 4 task was quite simple task as there was already examples, I can just load from the Arduino IDE. However, there was a slight twist, I had to modify the code slightly. This made me think carefully how am I going to modify the code, as I did not want get error when verifying the code. This made me understood the code even more and understood how each code works. One of the more challenging task was the modification of the melody code. I changed the components in the melody but there was a issue in the verification. So I can't transfer the code to uno maker kit, so I had to check what was the issue. The issue was that one of the tone in the melody was not defined in the pitches file, but further inspection found that the tone was already defined in the pitches file. Hence, I was very dumbfounded and did not know what to do. After a few minutes of troubleshooting, I had to delete the pitches file and install a new pitches file and upload it into the IDE. I verified the code and no issue came up.
After completing the pre-practical activity, I found myself better equipped for the practical day. The main activity was to construct a toy Pegasus that was aesthetic and was able to flap its wing with the help of the Arduino uno maker kit. As I was more familiar with the coding part, I was assigned the part where I had to come up with a code that was able to move the Pegasus wings. This was where the servo came in. I tried to be unique where I also included the programmable button into the code. However, I could not get it to work the way I wanted it to and after spending too much time trying to get the code to work. I decided that a melody would be our unique selling point instead. So, I decided to look up codes of songs that were appealing to me. I found a song called "Bohemian Rhapsody" so I decided to use that code and use it as part of our Pegasus toy. Another thing that I did not knew was that if you plug the jumper cable that was connected to the servo to the pin where the melody buzzer is playing, the servo will actually follow the tone of the melody. I found that pretty cool and decided to add that to our Pegasus toy.
Overall, this practical was challenging yet fun experience for me. It also taught me to a whole new skills that can set me aside from many of my peers from different schools. It also taught me that perseverance, as no matter how many times your codes fails, do not give up, look for different approaches and solutions. I believe that these lessons I learnt will be helpful in future endeavors.
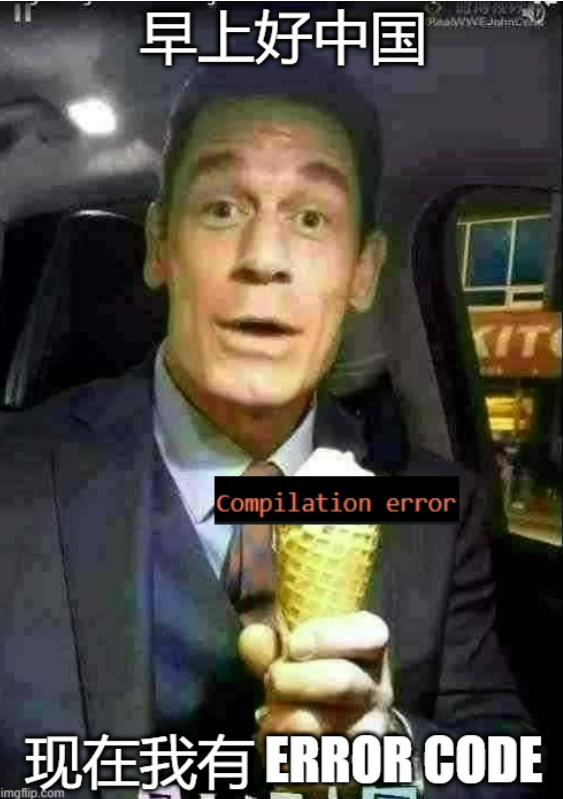
コメント